How to create a custom dialog in WiX for user input
During the installation process, user input dialogs play a crucial role by prompting users to provide necessary input. This feature is widely utilized in setup packages as it allows users to provide feedback, and it ensures proper installation and configuration of the software. Additionally, it allows you to store valuable information on the local machine for future use.
Let's say you have an application, and you want to create an installer package that collects information from the user.
In this tutorial, we’ll show you how to create a custom dialog to let the user provide input. We'll set it up so that it gets saved in a txt file on the local machine after installing the application.
To achieve this, let's now directly follow the steps outlined in the upcoming sections.
How to add the setup project?
To add the setup project for your application, go to File → New → Project. From the templates list, choose Setup Project for Wix.
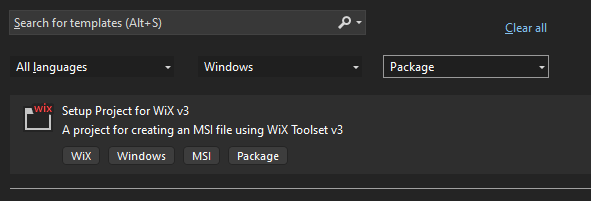
Keep in mind that you need to have the WiX extension added to Visual Studio to find the template. Check this tutorial to see how to add it. There, you’ll also find how to add the deployable files once the Setup Project is created.
Once you add the WiX extension and the deployable files, you have to edit the Product.wxs file and add the next line to reference the UI element we will use in this tutorial.
<Product Id = “*” … > <UIRef Id="CustomSetupUI" /> </Product>
How to create the installer dialogs?
Now, we are ready to create the dialogs for our installer package. A dialog is a Windows Installer XML file. We will create two dialogs for our installer package:
- A welcome dialog → SetupDlg.wxs
- A dialog for the user input → InputDlg.wxs
To create a dialog, follow the next steps:
- Right-click on the Setup Project → Add → New Item.
- Go to the WiX section and choose Installer File.
- Name it and click the Add button.
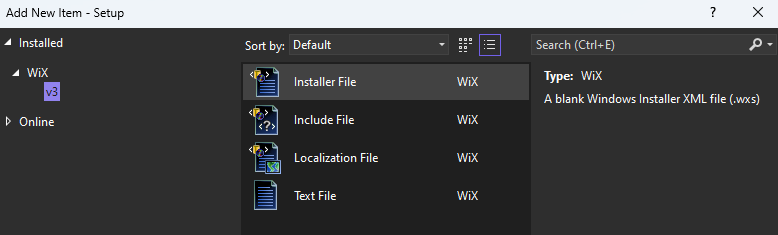
After you create the two dialogs, you should find them in the Solution Explorer under the Setup Project.
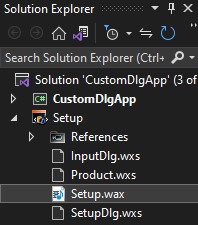
How to customize the installer dialogs?
Once the installer dialogs are created, you have to add the controls.
1. The welcome dialog from our example includes:
- Static texts
- Two buttons: Cancel (cancel the setup) and Next (proceed to the next dialog)
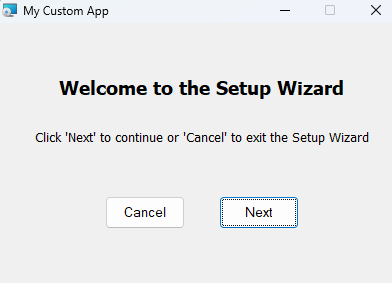
For this dialog, you can use the following code.
<?xml version="1.0" encoding="UTF-8"?> <Wix xmlns="http://schemas.microsoft.com/wix/2006/wi"> <Fragment> <UI Id="CustomSetupUI"> <TextStyle Id="RobotoBold" FaceName="RobotoBold" Size="10" /> <Property Id="DefaultUIFont" Value="RobotoBold" /> <DialogRef Id="InputDlg"/> <TextStyle Id="TahomaWelcome" FaceName="Tahoma" Size="14" Bold="yes" /> <TextStyle Id="TahomaInput" FaceName="Tahoma" Size="10" /> <Dialog Id="SetupDlg" Width="300" Height="200" Title="My Custom App"> <Control Id="WelcomeMsg" Type="Text" X="45" Y="40" Width="250" Height="80" Transparent="yes" Text="{\TahomaWelcome}Welcome to the Setup Wizard"/> <Control Id="Info" Type="Text" X="27" Y="80" Width="300" Height="80" Transparent="yes" Text="{\TahomaInfo}Click 'Next' to continue or 'Cancel' to exit the Setup Wizard"/> <Control Id="InstallBtn" Type="PushButton" Text="Next" Height ="25" Width="60" X="165" Y="130"> <Publish Event="EndDialog" Value="Return" /> </Control> <Control Id="CancelBtn" Type="PushButton" Text="Cancel" Height ="25" Width="60" X="80" Y="130" Cancel ="yes"> <Publish Event="EndDialog" Value="Exit" /> </Control> </Dialog> </UI> <InstallUISequence> <Show Dialog ="SetupDlg" Before="ExecuteAction"/> </InstallUISequence> </Fragment> </Wix>
2. The input dialog includes:
- Static texts
- Two edit boxes for the user input
- Two buttons: Cancel (cancel the installer) and Install (will start installing the application)
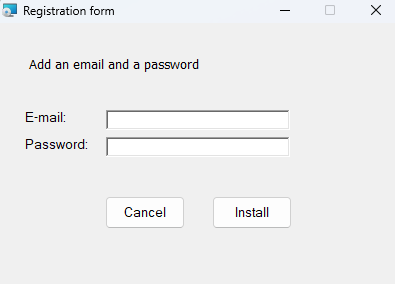
For this dialog, add the code below.
<?xml version="1.0" encoding="UTF-8"?> <Wix xmlns="http://schemas.microsoft.com/wix/2006/wi"> <Fragment> <UI Id="InputDlgUI"> <Dialog Id="InputDlg" Height="200" Width="300" Title="Registration form"> <Control Id="headerText" Type="Text" Height="50" Width="400" X="0" Y="0" TabSkip="no" /> <Control Id="RegistMsg" Type="Text" X="20" Y="25" Width="250" Height="80" Transparent="yes" Text="{\TahomaInfo} Add an email and a password"/> <Control Id="nameLabel" Type="Text" X="20" Y="65" Height="17" Width="65" Transparent="yes" Text="E-mail:" /> <Control Id="emailTextbox" Type="Edit" X="80" Y="65" Height="17" Width="140" Property="EMAIL" /> <Control Id="emailLabel" Type="Text" X="20" Y="85" Height="17" Width="65" Transparent="yes" Text="Password:" /> <Control Id="passwordTextbox" Type="Edit" X="80" Y="85" Height="17" Width="140" Property="PASSWORD" /> <Control Id="InstallBtn" Type="PushButton" Text="Install" Height ="25" Width="60" X="160" Y="130"> <Publish Event="DoAction" Value="UserInputCA">1</Publish> <Publish Event="EndDialog" Value="Return">1</Publish> </Control> <Control Id="CancelBtn" Type="PushButton" Text="Cancel" Height ="25" Width="60" X="80" Y="130" Cancel ="yes"> <Publish Event="EndDialog" Value="Exit" /> </Control> </Dialog> </UI> <InstallUISequence> <Show Dialog="InputDlg" After="SetupDlg" /> </InstallUISequence> </Fragment> <Fragment> <Binary Id="CustomActionBinary" SourceFile="$(var.UserInputCA.TargetDir)$(var.UserInputCA.TargetName).CA.dll"/> <CustomAction Id="UserInputCA" BinaryKey="CustomActionBinary" DllEntry="SaveUserInput" /> </Fragment> </Wix>
How to save the user input in a txt file?
Now, we have to take the information from the input dialog and save it in the txt file. For this, we will use a custom action. To create it, follow the steps:
1. Go to Files → New → Project and search for C# Custom Action Project for WiX.
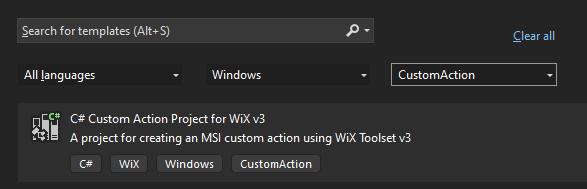
2. Next, add the following code to create the custom action:
public class CustomActions { [CustomAction] public static ActionResult SaveUserInput(Session session) { string email = session["EMAIL"]; string password = session["PASSWORD"]; string appdataPath = "C:\\Users\\Caphyon"; if (!Directory.Exists(appdataPath + "\\UserData")) Directory.CreateDirectory(appdataPath + "\\UserData"); File.WriteAllText(appdataPath + "\\UserData\\InputInfo.txt", email + "," + password); return ActionResult.Success; } }
As you can see, in order to store the user information, the custom action uses the Property attribute values of the emailTextBox and passwordTextBox control elements. The collected information will be written to a text file called InputInfo.txt and saved in the directory C:\Users\Caphyon\UserData.
3. After creating the custom action, you need to reference it in the Setup Project.
To do this, right-click on the Setup Project → Add → Reference. In the Add Reference dialog, go to the Projects tab and select the custom action that you created previously. Press the Add button and then the Ok button.
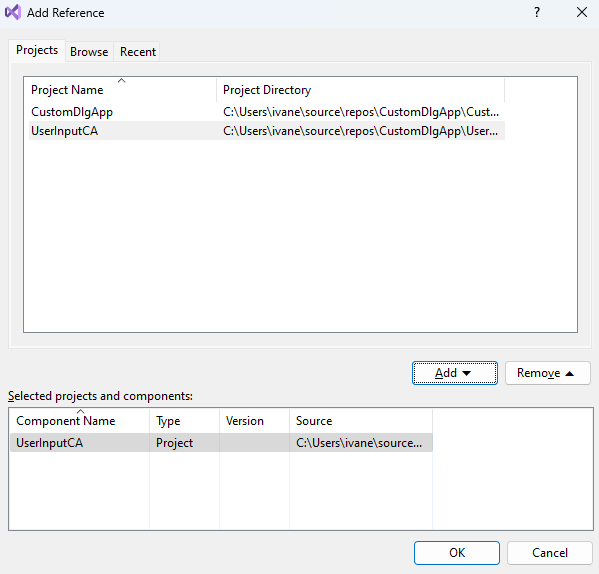
Build and install the project
After completing all the above steps, all you have to do is build the project and run the installer package.
Enter the data into the text fields you added to the input dialog and click on the install button. After the installation is complete, you should be able to locate the text file that contains the information you entered in the text boxes.
Conclusion
Designing user input dialogs in WiX can be challenging, particularly for complex scenarios like client-type applications. These cases often involve gathering and storing essential information, such as server address, port number, and firewall settings, in the registry. Editing the .wxs files to incorporate all the controls and create custom actions can be time-consuming.
A more streamlined and effective approach is to leverage a GUI-based tool. Advanced Installer provides an intuitive graphical user interface that simplifies the process of adding controls to dialogs. You can conveniently configure the controls directly from the user interface, saving you valuable time and effort.