License Key Validation in MSIX-Deployed WinForm Applications
With Microsoft's transition from MSI to MSIX, notable changes have emerged in application deployment, particularly in the software activation process.
Unlike the MSI format, where users could enter a license key during installation or via command line, MSIX shifts the activation phase and other configurations to the application's first launch.
This article will guide you through implementing license key activation support for a WinForm application deployed via MSIX.
We also have an article available on How to create an MSIX Package for a WinForms Application.
1. Creating the Application
First things first, let’s create a sample Windows Forms app in Visual Studio:
- Navigate to File → New → Project.
- Select the Windows Forms App template from the template list.
- Name and create your project.
2. Create the Licence Key Input Form
Once you’ve created the project, the next step is to develop a form for users to input the license key, including a text field for the key and an activation button.
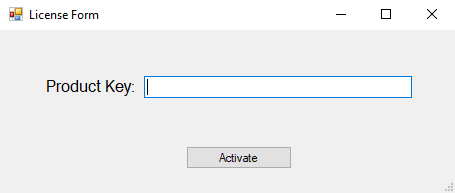
3. Check the License Key
Upon launch, the application checks if a valid license key exists in the registry. If not, it presents the license key input form. If the user exits without entering a key, the application verifies if it's within a 30-day trial period.
private const string registryPath = "HKEY_CURRENT_USER\\Your Company\\Your App"; private static bool CheckLicense() { var licenseKey = Registry.GetValue(registryPath, "LicenseKey", null) as string; if (licenseKey != null) { if (IsLicenseKeyValid(licenseKey)) { return true; } } var firstLaunchDate = Registry.GetValue(registryPath, "FirstLaunch", null); if (firstLaunchDate == null) { Registry.SetValue(registryPath, "FirstLaunch", DateTime.Now.ToString()); } else if (DateTime.Now > DateTime.Parse(firstLaunchDate.ToString()).AddDays(30)) { MessageBox.Show("Trial period has expired."); ShowLicenseForm(); licenseKey = Registry.GetValue(registryPath, "LicenseKey", null) as string; if (licenseKey != null && IsLicenseKeyValid(licenseKey)) { return true; } else { MessageBox.Show("Invalid license key. Exiting application."); Application.Exit(); return false; } } ShowLicenseForm(); return true; }
Before prompting users for the license key, the application checks for an internet connection. If unavailable, it informs the users that activation requires an internet connection.
private static void ShowLicenseForm() { if (!IsInternetAvailable()) { MessageBox.Show("An internet connection is required to activate the application."); return; } var licenseForm = new LicenseForm(); if (licenseForm.ShowDialog() == DialogResult.OK) { var enteredKey = licenseForm.productKey; bool isValidKey = IsLicenseKeyValid(enteredKey); if (isValidKey) { Registry.SetValue(registryPath, "LicenseKey", enteredKey); } else { MessageBox.Show("Invalid license key."); } } else { Application.Exit(); } } private static bool IsInternetAvailable() { try { using (var ping = new Ping()) { var reply = ping.Send("8.8.8.8", 3000); return reply.Status == IPStatus.Success; } } catch { return false; } }
4. Validating the License Key
When the user inputs their license key in the form, we have to verify it. For this purpose, we've chosen to keep a list of valid license keys in a text file on Dropbox, with each key on its own line. We then compare the key provided by the user against this list.
private static bool IsLicenseKeyValid(string licenseKey) { using (var client = new HttpClient()) { var responseTask = client.GetAsync(dropboxKeysFileUrl); var response = responseTask.Result; if (response.IsSuccessStatusCode) { var contentTask = response.Content.ReadAsStringAsync(); var content = contentTask.Result; using (var reader = new StringReader(content)) { string line; while ((line = reader.ReadLine()) != null) { if (line.Trim() == licenseKey) { return true; } } } } } return false; }
5. Package the app as an MSIX
The last step involves creating the MSIX package. You can do this using the Advanced Installer extension for Visual Studio, which is available in the Visual Studio marketplace. For help with using the extension to build the MSIX package, check out the instructions in the article below (video tutorial available):
6. Install and test the application
Once the package is created, run it to install the application. At the first launch, you will receive a prompt to activate the app. If you do not activate it, you will have a trial period of 30 days. During this period, you will be prompted to enter a license key every time you open the app.
Conclusion
Transitioning to MSIX offers a more streamlined and user-friendly deployment experience. By implementing the outlined steps, developers can efficiently integrate license key validation into their WinForm applications. This ensures a secure and compliant software activation process, aligning with the modern standards of application deployment.
Stay Ahead with Advanced Installer
Don't miss out on the latest tips, tools, and trends in software deployment.
Get exclusive insights, updates, and more delivered straight to your inbox.