How to set a Windows registry value with PowerShell
Navigating the Windows Registry can seem like wandering through a digital jungle. Fear not! Advanced Installer offers a user-friendly way to tweak registry keys and values.But what if you have a Custom Action in PowerShell or are a fan of the PowerShell App Deployment Toolkit (PSADT)? You might want to set registry key values using these tools.
Here's how.
What is Windows Registry?
Let's get our basics straight before diving in. The Windows Registry is the nerve center of your Windows operating system and the apps that call it home. It's a database storing vital configuration info.
Meddling in the Registry without care is like juggling chainsaws — thrilling but risky!
The Registry is a maze of keys within keys, storing values with specific data types. It's a flexible repository for system and application settings, organized into 'hives' — logical groupings that Windows loads during startup.
Remember the days when editing the Registry was a daredevil's game, done through regedit.exe?
Windows NT changed the game with the reg.exe command, ushering in safer programmatic management. And let's not forget WMI (Windows Management Instrumentation), another powerful tool for Registry wrangling.
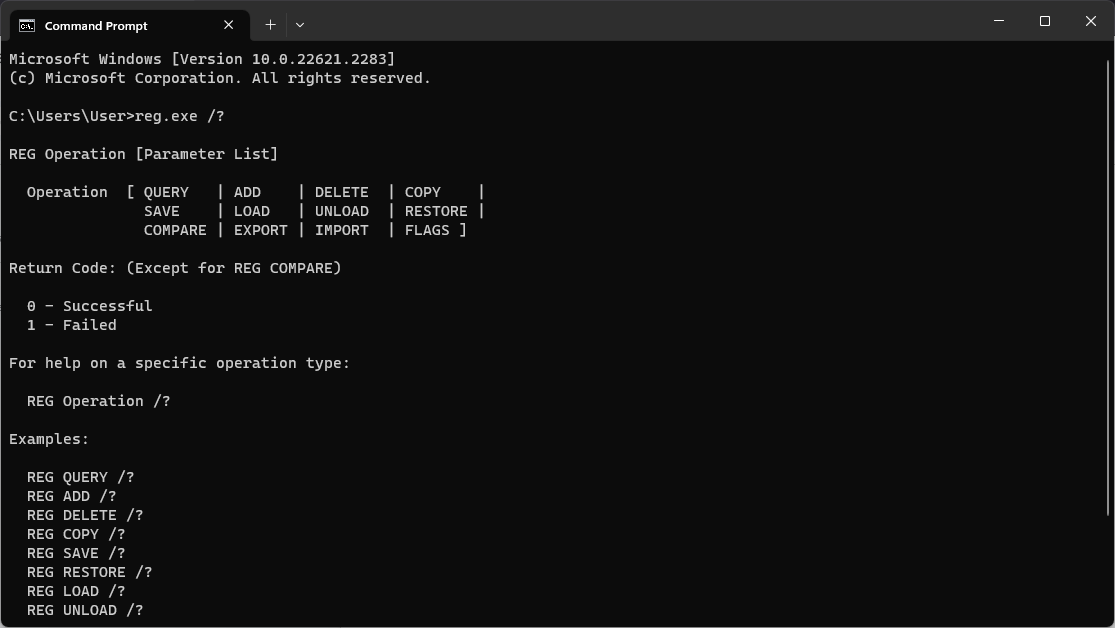
How to Set a Registry Key with PowerShell
For IT professionals working with PowerShell, life got easier with the introduction of the Registry provider. This nifty tool simplifies Registry tasks, transforming complex chores into a walk in the park.
PowerShell could have gone the route of creating a zillion cmdlets for every data store — Registry, files, certificates, you name it.
But instead, it introduced providers, an intermediary layer that turns these data stores into something akin to a file store. You can use familiar commands across different data types, thanks to these providers.
Curious about available providers? Run the `Get-PSProvider` cmdlet. It'll introduce you to:
- Registry: This provider deals with the Windows Registry, offering access to keys like HKLM (local machine) and HKCU (current user).
- Alias, Environment, and Function: These providers handle aliases, environment variables, and functions.
- FileSystem: This one's for managing files and folders.
- Certificate: For working with certificates.
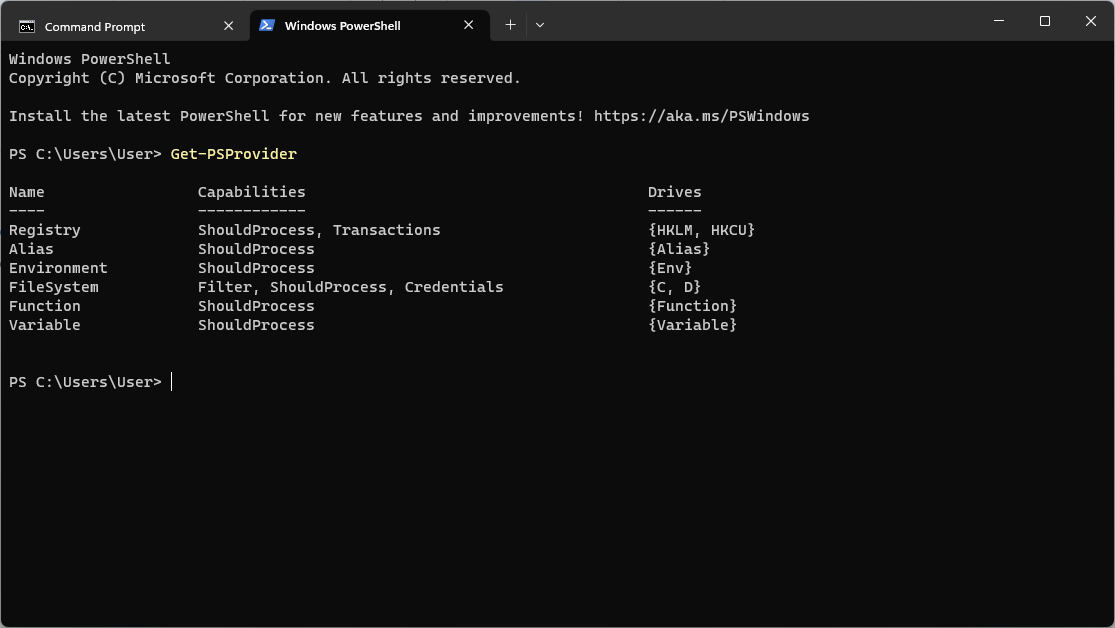
Providers create virtual drives, making it easier to access parts of these data stores. For example, the Registry provider creates drives like HKLM: (local machine) and HKCU: (current user) for easy access to Registry hives.
Registry Value Entries: The Building Blocks
Let's explore Registry Value Entries and how they play a role in PowerShell scripting.
Think of Registry Value Entries as attributes of registry keys. PowerShell's *-ItemProperty cmdlets are your tools for managing these.
Here's how you can use them in real-world scenarios:
Scenario 1
Imagine creating a "Version" registry value under the key "HKCU:\Software\Test\MyKey" with a value of "12".
You might be tempted to use the `New-ItemProperty` cmdlet like this:
$RegistryPath = 'HKCU:\Software\Test\MyKey’ $Name = 'Version' $Value = ‘12’ New-ItemProperty -Path $RegistryPath -Name $Name -Value $Value -PropertyType DWORD -Force
But if "HKCU:\Software\Test\MyKey" doesn't exist, you'll hit an error.
To avoid this, use Test-Path to check for the key's existence, and New-Item to create it if needed:
New-ItemProperty : Cannot find path 'HKCU:\Software\Test\MyKey' because it does not exist.
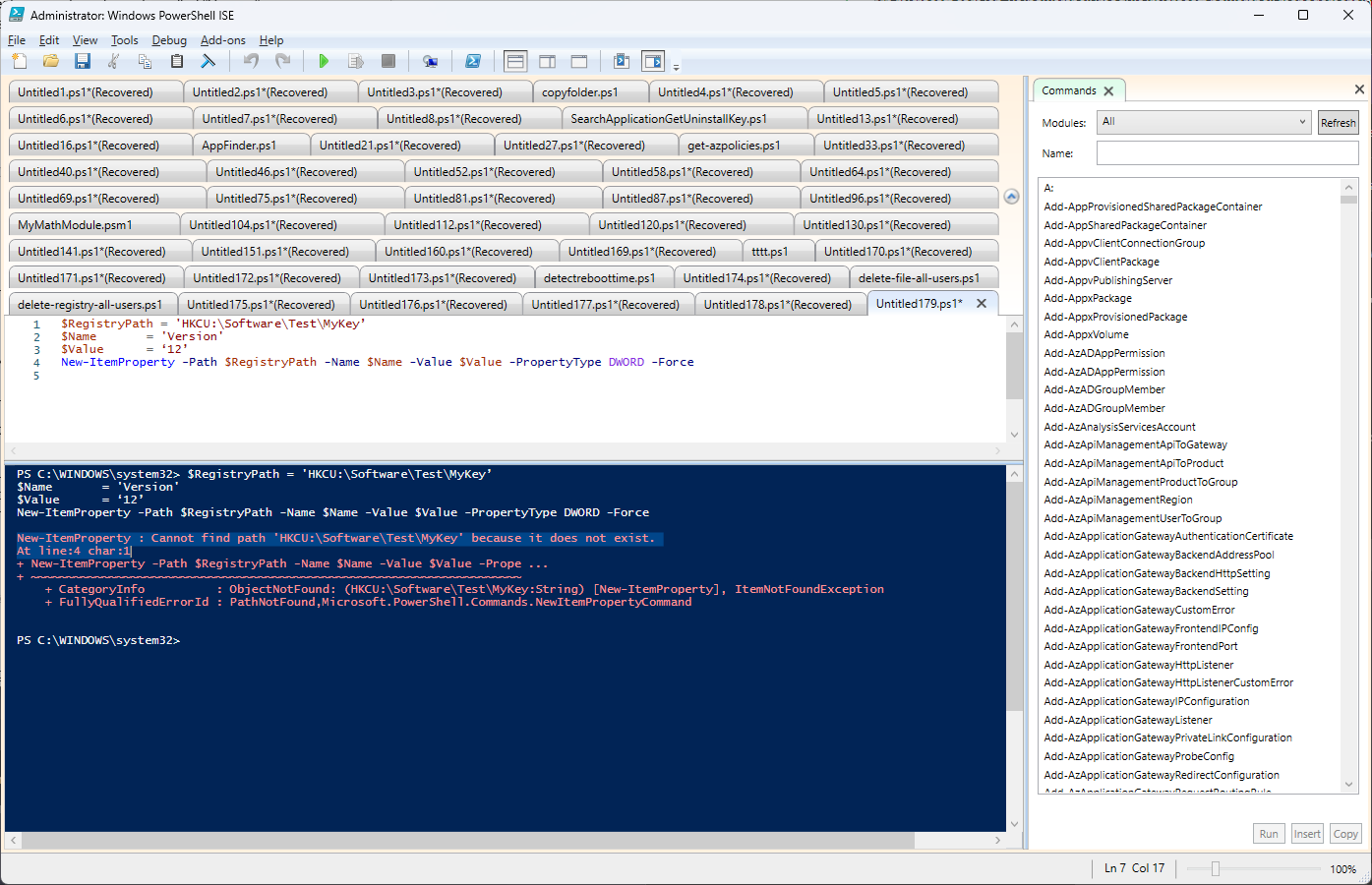
To address this issue more gracefully, you can take a safer approach:
# Set variables to indicate value and key to set $RegistryPath = 'HKCU:\Software\Test\MyKey’ $Name = 'Version' $Value = '12' # Create the key if it does not exist If (-NOT (Test-Path $RegistryPath)) { New-Item -Path $RegistryPath -Force | Out-Null } # Now set the value New-ItemProperty -Path $RegistryPath -Name $Name -Value $Value -PropertyType DWORD -Force
This modified script first checks whether the registry key exists using `Test-Path`. If it doesn't exist, it creates the key using `New-Item`. This way, you ensure that the required registry structure is in place before setting the value entry.
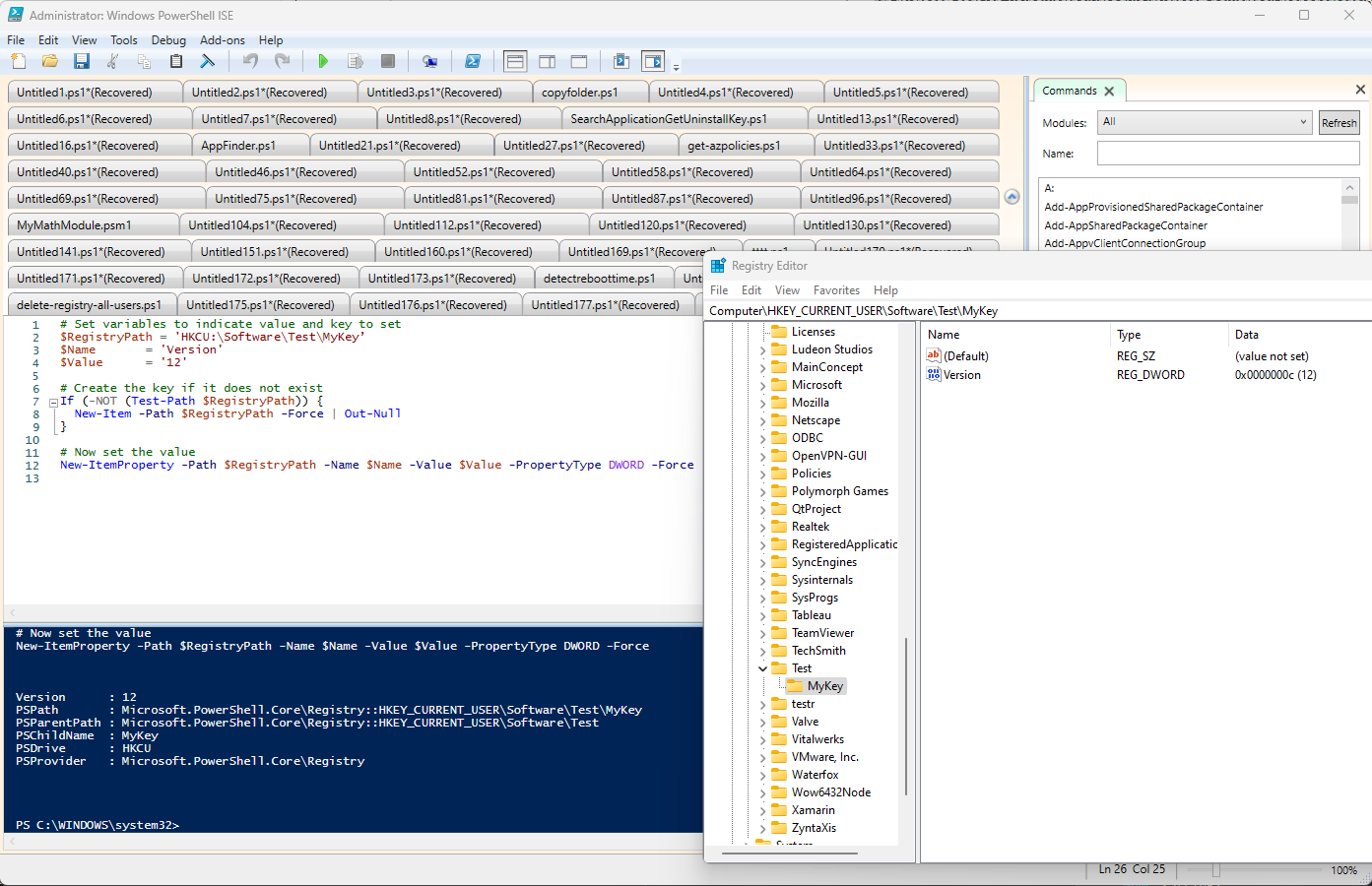
If we know that the registry key value already exists and we want to modify the value, we can use the `Set-ItemProperty` cmdlet. Think of each Registry Value Entry as an attribute of a particular registry key.
Scenario 2
Imagine you have a scenario where you want to update an existing registry value entry called "Version" under the key "HKCU:\Software\CommunityBlog\Scripts" with a new value, let's say "13."
You can achieve this using the `Set-ItemProperty` cmdlet. Here's an example of how you can do it:
# Define the registry path and property name $RegistryPath = 'HKCU:\Software\Test\MyKey’ $PropertyName = 'Version' # Specify the new value $NewValue = '13' # Use Set-ItemProperty to update the registry value Set-ItemProperty -Path $RegistryPath -Name $PropertyName -Value $NewValue
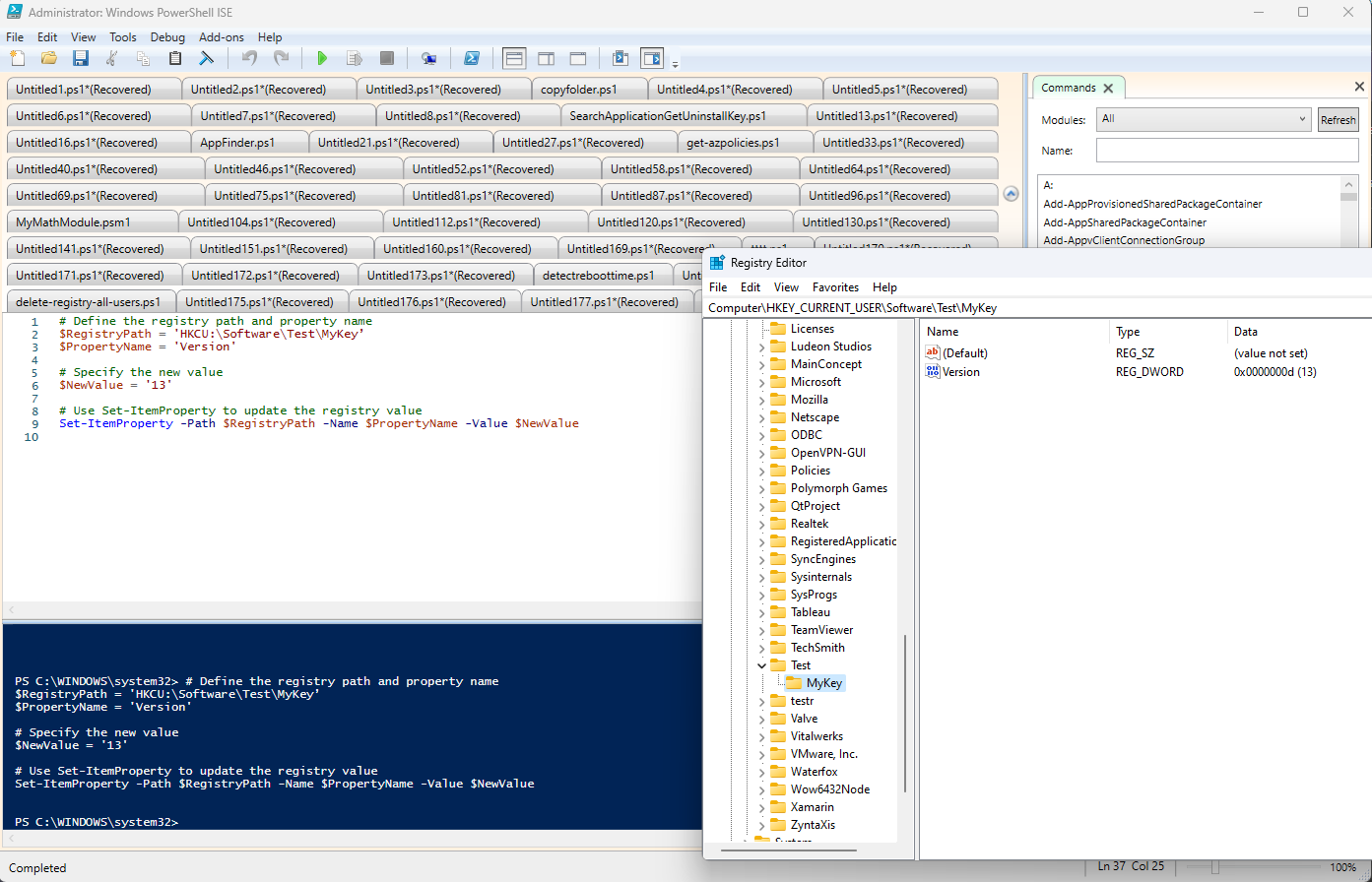
By running this script, you can effectively change the "Version" value in the specified registry key to "13."
This demonstrates how `Set-ItemProperty` can be a valuable tool for managing and updating registry values within your PowerShell scripts.
Tinkering with the registry, whether through the Registry Editor or PowerShell commands, can have serious consequences if done improperly.
Always exercise caution, back up critical data, and be certain of the changes you're making. The power of the registry comes with the responsibility to use it wisely!
Set a registry key with PowerShell App Deployment Toolkit
Now, for the IT pros responsible for getting software up and running on a bunch of Windows computers. Sometimes, you need to tweak things behind the scenes to make sure the software behaves just right. That's where the `Set-RegistryKey` function steps in.
This custom cmdlet makes it much easier to work with registry keys as opposed to the example we just had a look above. With this you don’t need to worry about additional checks in the registry by using Test-Path cmdlet.
This handy tool lets you tinker with the Windows Registry, a database that holds all sorts of settings for your operating system and applications. With `Set-RegistryKey`, you can make changes, create new settings, or fine-tune existing ones to match your needs.
Picture this: you're deploying a new app, and it's got a particular setting that needs to be just so. You can use `Set-RegistryKey` to make sure that setting is dialed in perfectly on all the computers you're working with. No need to manually mess around in the Registry – it's all automated.
Here's a quick example of how you might use it:
Let's say you're deploying a new application, and it needs a specific registry setting to work correctly. You can use `Set-RegistryKey` to create that setting and make sure it's set to the right value. No fuss, no manual Registry editing – it's all taken care of.
# Set a registry value to configure application behavior Set-RegistryKey -Key 'HKLM\Software\YourApplication' -Name 'SettingName' -Value 'DesiredValue' # Create a new registry key if it doesn't exist Set-RegistryKey -Key 'HKLM\Software\YourApplication\NewKey' # Modify an existing registry value Set-RegistryKey -Key 'HKCU\Software\YourApplication' -Name 'ExistingSetting' -Value 'UpdatedValue'
Conclusion
In essence, the Set-RegistryKey cmdlet in the PowerShell Application Deployment Toolkit is your secret weapon for smooth software deployments. It's like having a magic wand for the Windows Registry, ensuring your software runs seamlessly on every computer.
Happy scripting!